Feed-Forward Neural Network#
import numpy as np
import matplotlib.pyplot as plt
# n = 10
# np.ramdom.seed(0)
# x1 = np.random.uniform(0, 1, size=n)
# x2 = np.random.uniform(0, 1, size=n)
def xor(x1, x2):
return 1 * ((x1 + x2) == 1)
x1 = np.array([0, 1, 0, 1])
x2 = np.array([0, 0, 1, 1])
y = [xor(x1_, x2_) for x1_, x2_ in zip(x1, x2)]
for y_i in np.unique(y):
idx = y == y_i
plt.scatter(x1[idx], x2[idx], label=f"y = {y_i}")
plt.legend()
<matplotlib.legend.Legend at 0x7f85551c59f0>
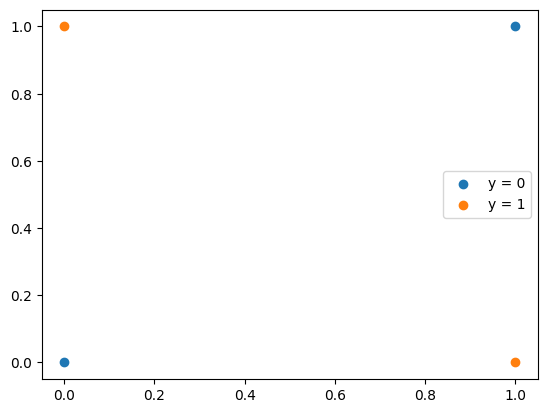
X = np.array([x1, x2]).T
X
array([[0, 0],
[1, 0],
[0, 1],
[1, 1]])
def step_function(x):
return np.array(x > 0)
def sigmoid(x):
return 1 / (1 + np.exp(-x))
def relu(x):
return np.maximum(0, x)
x = np.arange(-2, 2, .1)
plt.plot(x, step_function(x), label="step")
plt.plot(x, sigmoid(x), label="sigmoid")
plt.plot(x, relu(x), label="relu")
[<matplotlib.lines.Line2D at 0x7f8540407670>]
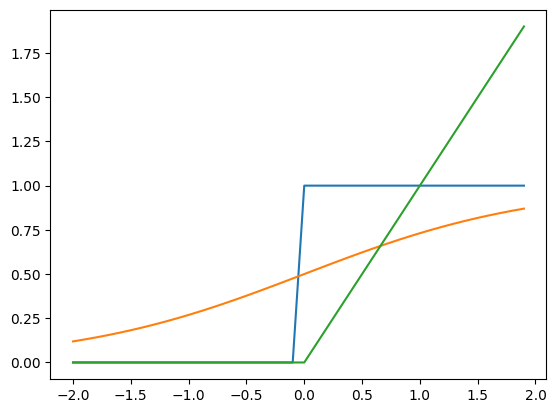
W = np.array([[1, 3, 5], [2, 4, 6]])
X @ W
array([[ 0, 0, 0],
[ 1, 3, 5],
[ 2, 4, 6],
[ 3, 7, 11]])
def identity_function(x):
return x
def init_network():
network = {}
network['W1'] = np.array([[0.1, 0.3, 0.5], [0.2, 0.4, 0.6]])
network['b1'] = np.array([0.1, 0.2, 0.3])
network['W2'] = np.array([[0.1, 0.4], [0.2, 0.5], [0.3, 0.6]])
network['b2'] = np.array([0.1, 0.2])
network['W3'] = np.array([[0.1, 0.3], [0.2, 0.4]])
network['b3'] = np.array([0.1, 0.2])
return network
def forward(network, x):
W1, W2, W3 = network['W1'], network['W2'], network['W3']
b1, b2, b3 = network['b1'], network['b2'], network['b3']
a1 = np.dot(x, W1) + b1
z1 = sigmoid(a1)
a2 = np.dot(z1, W2) + b2
z2 = sigmoid(a2)
a3 = np.dot(z2, W3) + b3
y = identity_function(a3)
return y
network = init_network()
x = np.array([1.0, 0.5])
y = forward(network, x)
print(y) # [ 0.31682708 0.69627909]
[0.31682708 0.69627909]
W1, W2, W3 = network['W1'], network['W2'], network['W3']
b1, b2, b3 = network['b1'], network['b2'], network['b3']
a1 = np.dot(x, W1) + b1
z1 = sigmoid(a1)
a2 = np.dot(z1, W2) + b2
z2 = sigmoid(a2)
a3 = np.dot(z2, W3) + b3
y = identity_function(a3)
a1 = x @ W1 + b1
z1 = sigmoid(a1)
z1
array([0.57444252, 0.66818777, 0.75026011])
Holyoak, K. J. (1987). Parallel distributed processing: explorations in the microstructure of cognition. Science, 236, 992-997.
Cell In[8], line 1
Holyoak, K. J. (1987). Parallel distributed processing: explorations in the microstructure of cognition. Science, 236, 992-997.
^
SyntaxError: invalid syntax